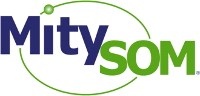 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
12 #include <libfpga/fpgaregister.h>
46 void enable(
bool abEnable);
@ eeRedBlue_Horizontal
Definition: Conv5x5.h:37
bool initialized()
Definition: Conv5x5.cpp:46
@ eeMono_Horizontal
Definition: Conv5x5.h:33
int setGaussianWeightsBayer(float sigma)
Definition: Conv5x5.h:66
@ eeGreen_Vertical
Definition: Conv5x5.h:36
teWeights
Definition: Conv5x5.h:32
int setWeight(teWeights aeWeights, int index, float weight)
Definition: Conv5x5.cpp:93
int setGaussianWeightsMono(float sigmaX, float sigmaY)
Definition: Conv5x5.cpp:260
@ eeGreen_Horizontal
Definition: Conv5x5.h:35
int setGaussianWeightsBayer(float sigmaXY_G, float sigmal_XY_RB)
Definition: Conv5x5.h:62
int setGaussianWeightsBayer(float sigmaX_G, float sigmaY_G, float sigmaX_RB, float sigmaY_RB)
Definition: Conv5x5.cpp:279
int setPassThroughWeights(void)
Definition: Conv5x5.cpp:184
int setGaussianWeightsMono(float sigmaXY)
Definition: Conv5x5.h:56
void setOperatingMode(teOperatingMode aeMode)
Definition: Conv5x5.cpp:68
virtual ~tcConv5x5()
Definition: Conv5x5.cpp:39
@ eeBayerModeGreenSecond
Definition: Conv5x5.h:28
int setWeights(teWeights aeWeights, const std::vector< float > &avWeights)
Definition: Conv5x5.cpp:137
@ eeMono_Vertical
Definition: Conv5x5.h:34
tcConv5x5(int anAddress)
Definition: Conv5x5.cpp:29
tcFPGARegister< uint32_t > mcReg
Definition: Conv5x5.h:75
teOperatingMode
Definition: Conv5x5.h:25
void enable(bool abEnable)
Definition: Conv5x5.cpp:55
static std::vector< float > Gaussian(float std_dev, teWeights weight_type)
Definition: Conv5x5.cpp:202
@ eeBayerModeGreenFirst
Definition: Conv5x5.h:27
@ eeMonochrome
Definition: Conv5x5.h:26
@ eeRedBlue_Vertical
Definition: Conv5x5.h:38