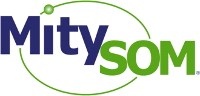 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file. 1 #ifndef tcDspParserBase_H
2 #define tcDspParserBase_H
6 #define WIN32_LEAN_AND_MEAN
14 #define INIT_CRITICAL(sema) InitializeCriticalSection(&(sema))
17 #define DEL_CRITICAL(sema) DeleteCriticalSection(&(sema))
19 #ifndef ENTER_CRITICAL
20 #define ENTER_CRITICAL(sema) EnterCriticalSection(&(sema))
22 #ifndef LEAVE_CRITICAL
23 #define LEAVE_CRITICAL(sema) LeaveCriticalSection(&(sema))
25 typedef CRITICAL_SECTION
C_SECT;
28 #elif defined(MITYDSP)
34 #define INIT_CRITICAL(sema) ((sema) = SEM_create(1, NULL))
37 #define DEL_CRITICAL(sema) (SEM_delete(sema))
39 #ifndef ENTER_CRITICAL
40 #define ENTER_CRITICAL(sema) (SEM_pend((sema), SYS_FOREVER))
42 #ifndef LEAVE_CRITICAL
43 #define LEAVE_CRITICAL(sema) (SEM_post(sema))
50 #define INIT_CRITICAL(sema)
53 #define DEL_CRITICAL(sema)
55 #ifndef ENTER_CRITICAL
56 #define ENTER_CRITICAL(sema)
58 #ifndef LEAVE_CRITICAL
59 #define LEAVE_CRITICAL(sema)
114 virtual bool ResetParser(
char *apOutBuf = NULL,
int anOutBufLen = 0);
115 virtual int AddData(
void *apData,
int anLength);
117 void *apUser = NULL);
134 int AssembleLine(
void *apInput,
int anLength,
bool &arLineComplete);
@ eeParseSyncErr
data sync error
Definition: DspParserBase.h:87
unsigned int mnBadMsgs
Count of bad messages.
Definition: DspParserBase.h:125
int mnOutBufLen
Current bytes written to output.
Definition: DspParserBase.h:144
virtual bool ResetParser(char *apOutBuf=NULL, int anOutBufLen=0)
Definition: DspParserBase.cpp:94
@ eeParseTooBigErr
result too big for output buffer
Definition: DspParserBase.h:89
@ eeParseChecksumErr
checksum failure
Definition: DspParserBase.h:86
Definition: DspParserBase.h:78
@ eeParseBustedMsg
broken message (missing data)
Definition: DspParserBase.h:88
int mnLineBufLen
Length of line buffer.
Definition: DspParserBase.h:148
virtual int AddData(void *apData, int anLength)
Definition: DspParserBase.cpp:184
@ eeParseOK
success
Definition: DspParserBase.h:85
bool(* tfParserCallback)(int, void *, int, unsigned int, void *)
Definition: DspParserBase.h:104
char * mpOutBuf
Pointer to output storage.
Definition: DspParserBase.h:143
int mnLinePos
Working position in the line buffer.
Definition: DspParserBase.h:149
unsigned int mnBytesWritten
Bytes written to buffer.
Definition: DspParserBase.h:123
unsigned int mnBytesRead
Bytes passed to parser.
Definition: DspParserBase.h:122
void * mpUserParameter
User-provided parameter to callback.
Definition: DspParserBase.h:150
@ eeBaseLast
base class
Definition: DspParserBase.h:90
char AsciiHexDigitVal(char anHexdigit)
Definition: DspParserBase.cpp:334
Definition: CameraTypes.h:7
virtual void RegisterCallback(tfParserCallback afCallback, void *apUser=NULL)
Definition: DspParserBase.cpp:242
virtual ~tcDspParserBase()
Definition: DspParserBase.cpp:74
tcDspParserBase(char *apOutBuf=NULL, int anOutBufLen=4096)
Definition: DspParserBase.cpp:41
bool mbStorageCreated
Indicates whether out buf created.
Definition: DspParserBase.h:142
C_SECT mhMutex
Semaphore to serialize access.
Definition: DspParserBase.h:141
char * maLineBuffer
(or other entities) to parse.
Definition: DspParserBase.h:146
unsigned int mnChecksumErrs
Number of checksum errors.
Definition: DspParserBase.h:127
unsigned int mnGoodMsgs
Count of valid messages decoded.
Definition: DspParserBase.h:124
unsigned int mnChecksum
Storage to accumulate checksum.
Definition: DspParserBase.h:145
teDspParseStatus
possible return status'
Definition: DspParserBase.h:83
int C_SECT
Definition: DspParserBase.h:61
unsigned int mnSyncErrs
Number of synchronization errors.
Definition: DspParserBase.h:126
int AssembleLine(void *apInput, int anLength, bool &arLineComplete)
Definition: DspParserBase.cpp:264
tfParserCallback mfCallback
Storage for parser callback.
Definition: DspParserBase.h:140