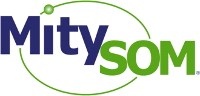 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
8 #ifndef __INIREADER_H__
9 #define __INIREADER_H__
28 std::string
Get(
const std::string& section,
const std::string& name,
29 const std::string& default_value)
const;
33 long GetInteger(
const std::string& section,
const std::string& name,
long default_value)
const;
37 unsigned long GetUnsignedInteger(
const std::string& section,
const std::string& name,
unsigned long default_value)
const;
42 double GetReal(
const std::string& section,
const std::string& name,
double default_value)
const;
47 bool GetBoolean(
const std::string& section,
const std::string& name,
bool default_value)
const;
51 std::map<std::string, std::string> _values;
52 static std::string MakeKey(
const std::string& section,
const std::string& name);
53 static int ValueHandler(
void* user,
const char* section,
const char* name,
57 #endif // __INIREADER_H__
long GetInteger(const std::string §ion, const std::string &name, long default_value) const
Definition: INIReader.cpp:33
double GetReal(const std::string §ion, const std::string &name, double default_value) const
Definition: INIReader.cpp:53
bool GetBoolean(const std::string §ion, const std::string &name, bool default_value) const
Definition: INIReader.cpp:62
int ParseError() const
Definition: INIReader.cpp:21
Definition: INIReader.h:16
unsigned long GetUnsignedInteger(const std::string §ion, const std::string &name, unsigned long default_value) const
Definition: INIReader.cpp:43
INIReader(const std::string &filename)
Definition: INIReader.cpp:16
std::string Get(const std::string §ion, const std::string &name, const std::string &default_value) const
Definition: INIReader.cpp:26