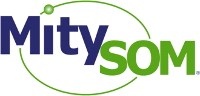 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
17 tcJob(
const char* apName);
37 const char*
getName()
const {
return mpName; }
63 + (arT.tv_nsec) / 1000000 ; }
73 std::ostream&
operator <<(std::ostream& arS,
const struct timespec& arT);
@ eeScheduled
Definition: Job.h:23
int doExecute()
run the job
Definition: Job.cpp:22
virtual uint64 getNextRunTimeMs() const
Definition: Job.cpp:70
uint64_t uint64
Definition: Types.h:13
virtual teStatus status() const
get the job status
Definition: Job.h:49
int mnPeriodMs
task period (ms)
Definition: Job.h:57
tcPeriodicJobThread * mpThread
thread job will run in
Definition: Job.h:55
teStatus meStatus
Definition: Job.h:33
void detachThread()
remove this job from its thread (if attached)
Definition: Job.cpp:47
uint64 mnLastRunMs
last run time
Definition: Job.h:59
teStatus
Definition: Job.h:20
virtual ~tcJob()
Definition: Job.cpp:12
@ eeRunning
Definition: Job.h:24
Definition: PeriodicJobThread.h:18
virtual int getPeriodMs() const
Get the period of this job (called by thread!)
Definition: Job.h:44
const char * getName() const
get the name of the job
Definition: Job.h:37
void attachThread(tcPeriodicJobThread *apThread)
attach this job to a thread
Definition: Job.cpp:35
void enterExecute()
convenience function for stats
Definition: Job.cpp:57
@ eeStopped
Definition: Job.h:22
std::ostream & operator<<(std::ostream &arS, const struct timespec &arT)
Definition: Job.cpp:92
tcJob(const char *apName)
Definition: Job.cpp:5
struct timespec msStopLast
Definition: Job.h:32
void exitExecute()
tcJob::exitExecute - called at the end of execute to update stats
Definition: Job.cpp:85
@ eeFailed
Definition: Job.h:25
struct timespec msStartLast
Definition: Job.h:31
virtual int execute()=0
run the job
uint64 timespecToMs(const struct timespec arT) const
Definition: Job.h:62
tcStats mcStats
job status
Definition: Job.h:61
The tcJob class is the base class for jobs that get added to the tcPeriodicJobThread.
Definition: Job.h:14