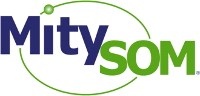 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
11 #include <libfpga/fpgaregister.h>
21 void reset(
bool abReset);
28 void setRow(uint32_t anStart, uint32_t anWidth);
40 int setCol(uint32_t anStart, uint32_t anHeight);
51 tcFPGARegister<uint32_t> mhRowAdjust;
int setColHeight(uint32_t anHeight)
Definition: RowAdjuster.cpp:170
uint32_t getColStart()
Definition: RowAdjuster.cpp:158
uint32_t getRowWidth()
Definition: RowAdjuster.cpp:112
bool initialized()
tcRowAdjuster::initialized
Definition: RowAdjuster.cpp:43
void reset(bool abReset)
Definition: RowAdjuster.cpp:52
void setRowWidth(uint32_t anWidth)
Definition: RowAdjuster.cpp:102
Definition: RowAdjuster.h:14
int enableYClip(bool abEnable)
Definition: RowAdjuster.cpp:289
bool canDecimateX(void)
Definition: RowAdjuster.cpp:217
void keepOddDecimatedSamples(bool abKeepOdd)
Definition: RowAdjuster.cpp:277
uint32_t getRowStart()
Definition: RowAdjuster.cpp:87
bool headerDecodeError(void)
Definition: RowAdjuster.cpp:247
int setCol(uint32_t anStart, uint32_t anHeight)
Definition: RowAdjuster.cpp:194
void dumpRegisters()
Definition: RowAdjuster.cpp:315
int enableDecimateX(bool abEnable)
Definition: RowAdjuster.cpp:257
void enableTestPattern(bool abEnable)
Definition: RowAdjuster.cpp:307
void setRowStart(uint32_t anStart)
Definition: RowAdjuster.cpp:77
bool canClipY(void)
Definition: RowAdjuster.cpp:226
virtual ~tcRowAdjuster()
Definition: RowAdjuster.cpp:36
void setRow(uint32_t anStart, uint32_t anWidth)
Definition: RowAdjuster.cpp:123
bool readReset()
tcRowAdjuster::readReset
Definition: RowAdjuster.cpp:65
uint32_t getColHeight()
Definition: RowAdjuster.cpp:182
uint8_t getPixelsPerClock(void)
Definition: RowAdjuster.cpp:235
tcRowAdjuster(uint32_t anAddress)
Definition: RowAdjuster.cpp:31
int setColStart(uint32_t anStart)
Definition: RowAdjuster.cpp:146