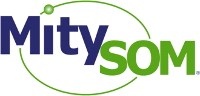 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file. 1 #ifndef STRINGTOKENIZER_H
2 #define STRINGTOKENIZER_H
91 std::string mhToTokenize;
92 std::istringstream mhStringStream;
95 static inline std::string <rim(std::string &s)
97 size_t num_spaces = 0;
98 while ((num_spaces < s.size()) && std::isspace(s.at(num_spaces))) {
101 s.erase(0,num_spaces);
106 static inline std::string &rtrim(std::string &s)
109 size_t num_spaces = 0;
110 size_t index = s.size() - 1;
111 while ((index > 0) && (num_spaces < s.size()) && std::isspace(s.at(index))) {
115 s.erase(index + 1, std::string::npos);
121 static inline std::string &trim(std::string &s)
123 return ltrim(rtrim(s));
128 #endif // STRINGTOKENIZER_H
bool nextUIntHex(uint32 *apRet)
Definition: StringTokenizer.cpp:45
int32_t int32
Definition: Types.h:8
bool nextFloat(float *apRet)
Definition: StringTokenizer.cpp:108
bool nextDouble(double *apRet)
Definition: StringTokenizer.cpp:92
bool nextIntHex(int32 *apRet)
Definition: StringTokenizer.cpp:29
tcStringTokenizer(std::string ahToTokenize, char ahDelimeter=' ')
Definition: StringTokenizer.cpp:4
bool nextUnsigned(uint32 *apRet)
Definition: StringTokenizer.cpp:76
bool hasNext()
Definition: StringTokenizer.cpp:13
Definition: StringTokenizer.h:14
uint32_t uint32
Definition: Types.h:11
bool nextInt(int32 *apRet)
Definition: StringTokenizer.cpp:60
std::string next()
Definition: StringTokenizer.cpp:18
bool nextBool(bool *apRet)
Definition: StringTokenizer.cpp:124