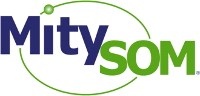 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
46 virtual void*
run() = 0;
62 static void* pthread_entry(
void* apSelf);
static const int HIGH_PRIORITY
Definition: Thread.h:11
virtual ~tcThread()
Definition: Thread.cpp:12
virtual void start()
Definition: Thread.cpp:21
static const int NORMAL_PRIORITY
Definition: Thread.h:12
tcThread()
Definition: Thread.cpp:6
static const int HIGHEST_PRIORITY
Definition: Thread.h:10
static const int LOWEST_PRIORITY
Definition: Thread.h:14
virtual void stop()
Definition: Thread.cpp:30
static const int LOW_PRIORITY
Definition: Thread.h:13
void setPriority(int anRelativePriority)
Definition: Thread.cpp:35
void join()
Definition: Thread.cpp:16
bool mbKillThread
Definition: Thread.h:56