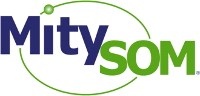 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
21 class tcU3VisionInterface;
38 void nack(tsU3VMsg* apMsg, uint16_t statusCode);
44 void log(tsU3VMsg* apMsg);
45 void sync(tsU3VMsg* apMsg);
46 void init(tsU3VMsg* apMsg);
47 void usbUpdate(tsU3VMsg* apMsg);
50 void readMem(tsU3VMsg* apMsg);
51 void writeMem(tsU3VMsg* apMsg);
52 void event(tsU3VMsg* apMsg);
55 void cpy_details(
void * apDest,
const INIReader &arIni,
const std::string& arName,
const std::string& arDefault);
58 uint16_t execWriteRegCmd(uint32_t address, uint8_t *data);
62 std::map< uint32_t, std::map<uint16_t, delFunc> > functions;
void nack(tsU3VMsg *apMsg, uint16_t statusCode)
Definition: U3VDelegate.cpp:419
void(tcU3VDelegate::* delFunc)(tsU3VMsg *apMsg)
Definition: U3VDelegate.h:24
delFunc getFunction(uint32_t prefix, uint16_t cmdId)
Definition: U3VDelegate.cpp:48
Definition: U3VisionInterface.h:25
Definition: U3VDelegate.h:31
Definition: INIReader.h:16
Definition: tcPendingAckWatchdog.h:31
tcU3VDelegate(tcU3VisionInterface *apIface)
Definition: U3VDelegate.cpp:28
Definition: CameraTypes.h:7
virtual void pendingAckNeeded(const void *id)
Definition: U3VDelegate.cpp:362
virtual ~tcU3VDelegate()
Definition: U3VDelegate.cpp:45