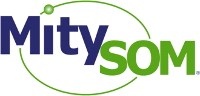 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
18 #define PVAR(v) " "#v" = " << (v)
21 #define PVARX(v) " "#v" = 0x" << std::hex << (v) << std::dec
24 #define PLOC __FILE__ << ":" << __LINE__ << " "
27 extern void str_replace (std::string &s1,
const std::string &s2,
const std::string &s3);
28 extern void str_remove (std::string &s1,
const std::string &s2);
30 extern void str_pad (std::string &s1,
int len,
char cc);
31 extern uint32_t
str_to_uint32 (
const std::string &s1,
int base = 10);
34 extern void str_vsprintf (std::string &s1,
const char *fmt, va_list args);
35 extern void str_sprintf (std::string &s1,
const char *fmt, ...);
36 extern std::string
str_sprintf (
const char *fmt, ...);
37 extern void str_split (std::vector<std::string>& list,
const std::string &s1,
const std::string &s2,
bool keep_empty =
true);
38 extern void str_strcpy (
char* destination,
const std::string& source,
size_t dest_buf_size);
44 template <
size_t dest_buf_size>
45 void str_strcpy (
char (&destination)[dest_buf_size],
const std::string& source)
47 str_strcpy(destination, source, dest_buf_size);
54 extern void str_from_ip (std::string &s1, uint32_t ip);
57 extern uint32_t
ip_from_str (
const std::string &s1);
59 extern void parse_macaddr (
const std::string &s1, uint8_t* macaddr);
61 extern std::string
xml_escape (
const char *str);
62 extern std::string
xml_escape (std::string str);
63 extern void split_ip (uint32_t ip, uint8_t *octets);
64 extern void form_ip (uint32_t &ip, uint8_t *octets);
69 void hexdump(
void *ptr,
int buflen);
uint32_t ip_from_str(const char *s1)
Definition: str_funcs.cpp:281
void str_length_trim(std::string &s1, int maxlen)
Definition: str_funcs.cpp:78
std::string fileToString(const char *filename)
Definition: str_funcs.cpp:387
void str_replace(std::string &s1, const std::string &s2, const std::string &s3)
Definition: str_funcs.cpp:39
void form_ip(uint32_t &ip, uint8_t *octets)
Converts four octets into a uint32 IP address.
Definition: str_funcs.cpp:376
uint32_t str_to_uint32(const std::string &s1, int base=10)
Definition: str_funcs.cpp:98
std::string xml_escape(const char *str)
Definition: str_funcs.cpp:313
void parse_macaddr(const std::string &s1, uint8_t *macaddr)
Definition: str_funcs.cpp:291
void str_sprintf(std::string &s1, const char *fmt,...)
Definition: str_funcs.cpp:174
std::string getcwd_string()
Get the cwd as a string object.
Definition: str_funcs.cpp:409
void str_tolower(std::string &s1)
Definition: str_funcs.cpp:116
void str_vsprintf(std::string &s1, const char *fmt, va_list args)
Definition: str_funcs.cpp:129
void str_toupper(std::string &s1)
Definition: str_funcs.cpp:103
void str_from_ip(std::string &s1, uint32_t ip)
Definition: str_funcs.cpp:268
void split_ip(uint32_t ip, uint8_t *octets)
Converts a uint32 IP address into four octets.
Definition: str_funcs.cpp:365
std::string format_macaddr(const uint8_t *macaddr)
Definition: str_funcs.cpp:304
void str_remove(std::string &s1, const std::string &s2)
Definition: str_funcs.cpp:73
std::string format_ipaddr(uint32_t ip)
Definition: str_funcs.cpp:274
void str_split(std::vector< std::string > &list, const std::string &s1, const std::string &s2, bool keep_empty=true)
Definition: str_funcs.cpp:213
void str_pad(std::string &s1, int len, char cc)
Definition: str_funcs.cpp:90
void str_strcpy(char *destination, const std::string &source, size_t dest_buf_size)
Definition: str_funcs.cpp:255
void hexdump(void *ptr, int buflen)
simple hex dump routine
Definition: str_funcs.cpp:422