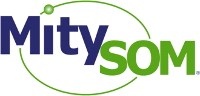 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
2 #ifndef tcCommandExecutiveBase_H_
3 #define tcCommandExecutiveBase_H_
18 #define MENU_FUNCTION_DECL(name) \
19 static void name (void* me) { ((tcCommandExecutiveBase*)me)-> name (); } \
63 bool ask_on_off (
const char *prompt,
bool allowempty =
false,
bool dflt =
false);
64 bool ask_yes_no (
const char *prompt,
bool allowempty =
false,
bool dflt =
false);
65 double ask_double (
const char *prompt,
bool allowempty =
false,
double dflt = 0);
66 float ask_float (
const char *prompt,
bool allowempty =
false,
float dflt = 0);
67 uint32_t
ask_uint (
const char *prompt,
bool allowempty =
false, uint32_t dflt = 0);
68 int32_t
ask_int (
const char *prompt,
bool allowempty =
false, int32_t dflt = 0);
69 std::string
ask_string (
const char *prompt,
bool allowempty =
false,
const char *dflt =
"");
95 #undef MENU_FUNCTION_DECL // We don't need this anymore
111 #endif // tcCommandExecutiveBase_H_
tcUIThread * m_ConsoleListenerThread
Thread handle for User Input.
Definition: tcCommandExecutiveBase.h:84
int32_t ask_int(const char *prompt, bool allowempty=false, int32_t dflt=0)
Definition: tcCommandExecutiveBase.cpp:182
tcUIThread(tcCommandExecutiveBase *exec)
Definition: tcCommandExecutiveBase.h:104
std::string m_appname
Definition: tcCommandExecutiveBase.h:87
void conditionalRead(unsigned int anTabs, const char *apMessage)
Definition: tcCommandExecutiveBase.cpp:281
std::string m_cmnd
Definition: tcCommandExecutiveBase.h:76
void printHelpAll() const
Definition: tcCommandExecutiveBase.cpp:368
tsCmndFunc * get_cmndfunc(const char *cmnd)
Definition: tcCommandExecutiveBase.cpp:58
This class forms the user input thread for the menu.
Definition: tcCommandExecutiveBase.h:101
bool ask_yes_no(const char *prompt, bool allowempty=false, bool dflt=false)
Generic "yes/no" asker.
Definition: tcCommandExecutiveBase.cpp:238
void start()
Definition: tcCommandExecutiveBase.cpp:88
static const int NORMAL_PRIORITY
Definition: Thread.h:12
Definition: tcCommandExecutiveBase.h:36
tpVoidFunc m_func
Definition: tcCommandExecutiveBase.h:77
float ask_float(const char *prompt, bool allowempty=false, float dflt=0)
Definition: tcCommandExecutiveBase.cpp:147
Definition: tcCommandExecutiveBase.h:74
bool m_continue
Tells the UI thread when QUIT command has been given.
Definition: tcCommandExecutiveBase.h:85
std::string ask_string(const char *prompt, bool allowempty=false, const char *dflt="")
Definition: tcCommandExecutiveBase.cpp:199
bool ask_on_off(const char *prompt, bool allowempty=false, bool dflt=false)
Generic "on/off" asker.
Definition: tcCommandExecutiveBase.cpp:216
void(* tpVoidFunc)(void *)
Definition: tcCommandExecutiveBase.h:43
void UserInputLoop()
Definition: tcCommandExecutiveBase.cpp:296
void add_seperator()
Definition: tcCommandExecutiveBase.cpp:52
void setPriority(int anRelativePriority)
Definition: Thread.cpp:35
tcCommandExecutiveBase * m_exec
Definition: tcCommandExecutiveBase.h:107
std::list< std::string > m_cmnd_list
Definition: tcCommandExecutiveBase.h:86
double ask_double(const char *prompt, bool allowempty=false, double dflt=0)
Definition: tcCommandExecutiveBase.cpp:130
tcCommandExecutiveBase(const char *appname)
Constructor, Create an input thread and then populate the menu and the help menu.
Definition: tcCommandExecutiveBase.cpp:13
void printHelp(const char *cmnd)
Prints either a single command, all commands, or an error, depending on the help string.
Definition: tcCommandExecutiveBase.cpp:335
std::vector< tsCmndFunc > m_cmnd_func_list
Definition: tcCommandExecutiveBase.h:80
tpVoidFunc get_func(const char *cmnd)
Definition: tcCommandExecutiveBase.cpp:74
uint32_t ask_uint(const char *prompt, bool allowempty=false, uint32_t dflt=0)
Definition: tcCommandExecutiveBase.cpp:164
void ReadUserInput()
Definition: tcCommandExecutiveBase.cpp:260
void * run()
Definition: tcCommandExecutiveBase.h:105
void printHelpGeneric(const tsCmndFunc *tcf) const
Prints generic formatted help for the specfied command and info.
Definition: tcCommandExecutiveBase.cpp:358
void add_cmnd(const char *cmnd, tpVoidFunc func, const char *desc)
Definition: tcCommandExecutiveBase.cpp:37
MENU_FUNCTION_DECL(process_help)
std::string m_help
Definition: tcCommandExecutiveBase.h:78