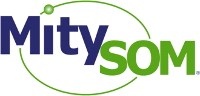 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
8 #ifndef TCU3VFX3CORE_H_
9 #define TCU3VFX3CORE_H_
13 #include <libfpga/fpgaregister.h>
22 void reset(
bool abReset);
23 void enable(
bool abEnable);
48 void stall(
bool abStall);
55 void setBit(
bool abSet, uint32_t anOffset, uint32_t anBit);
57 tcFPGARegister<uint32_t> mhRegister;
59 uint32_t mnSirmCount, mnSirmTs, mnSirmFt1, mnSirmFt2;
void enable(bool abEnable)
Definition: tcU3VFX3Core.cpp:59
void setInterPacketStall(int anNumberOfPackets, int anNumClockCycles)
Definition: tcU3VFX3Core.cpp:131
void setBytesPerFrame(uint32_t anNumBytes)
Definition: tcU3VFX3Core.cpp:116
void setOverrun(bool abSet)
Definition: tcU3VFX3Core.cpp:157
void setFrameOffsets(uint16_t anX, uint16_t anY)
Definition: tcU3VFX3Core.cpp:147
uint32_t version()
Definition: tcU3VFX3Core.cpp:49
void setFrameSize(uint16_t anX, uint16_t anY)
Definition: tcU3VFX3Core.cpp:142
void latchTimestamp()
Definition: tcU3VFX3Core.cpp:76
void setSIRMTransferSize(uint32_t anTransferSize)
Definition: tcU3VFX3Core.cpp:92
virtual ~tcU3VFX3Core()
Definition: tcU3VFX3Core.cpp:45
bool transferError()
Definition: tcU3VFX3Core.cpp:64
Definition: tcU3VFX3Core.h:15
void stall(bool abStall)
Definition: tcU3VFX3Core.cpp:121
void setPixelFormat(uint32_t anPixelFormat)
Definition: tcU3VFX3Core.cpp:137
uint64_t timestamp()
Definition: tcU3VFX3Core.cpp:81
void setSIRMFT2(uint32_t anFT2)
Definition: tcU3VFX3Core.cpp:110
void setSIRMFT1(uint32_t anFT1)
Definition: tcU3VFX3Core.cpp:104
void setSIRMTransferCount(uint32_t anTransferCount)
Definition: tcU3VFX3Core.cpp:98
bool isStalled()
Definition: tcU3VFX3Core.cpp:126
uint32_t SIRMBytes()
Definition: tcU3VFX3Core.cpp:152
tcU3VFX3Core(uint32_t anAddress)
Definition: tcU3VFX3Core.cpp:36
void reset(bool abReset)
Definition: tcU3VFX3Core.cpp:54