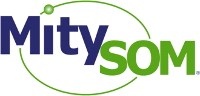 |
Critical Link MityCam SoC Firmware
1.0
Critical Link MityCam SoC Firmware
|
Go to the documentation of this file.
47 #ifndef REGISTERFILE_H
48 #define REGISTERFILE_H
55 #include <sys/types.h>
56 #include <sys/socket.h>
57 #include <netinet/in.h>
77 #define REG_ACCESS_NA 0,0 //<! Access mode NA - Not Available.
78 #define REG_ACCESS_WO 0,1 //<! Access mode WO - Write Only
79 #define REG_ACCESS_RO 1,0 //<! Access mode RO - Read Only
80 #define REG_ACCESS_RW 1,1 //<! Access mode RW - Readable & Writable
98 #define REGDEF_I64(name,access,dflt) { name ## _ADDR, #name, sizeof(uint64_t), SocCamera::eeRegUint64, REG_ACCESS_ ## access, { .dfltlval = dflt }, std::string() }
99 #define REGDEF_INT(name,access,dflt) { name ## _ADDR, #name, sizeof(uint32_t), SocCamera::eeRegUint32, REG_ACCESS_ ## access, { .dfltnval = dflt }, std::string() }
100 #define REGDEF_FLT(name,access,dflt) { name ## _ADDR, #name, sizeof(float), SocCamera::eeRegFloat, REG_ACCESS_ ## access, { .dfltfval = dflt }, std::string() }
101 #define REGDEF_STR(name,access,size,dflt) { name ## _ADDR, #name, size, SocCamera::eeRegString, REG_ACCESS_ ## access, { .dfltnval = 0 }, dflt }
102 #define REGDEF_BUF(name,access,size) { name ## _ADDR, #name, size, SocCamera::eeRegNoType, REG_ACCESS_ ## access, { .dfltrptr = nullptr }, std::string() }
103 #define REGDEF_END_OF_TABLE { 0xFFFFFFFF,NULL,0,SocCamera::eeRegUint32,0,0,{ .dfltnval = 0 },std::string() }
168 int get_array (uint32_t address, uint8_t* buff, uint16_t length)
const;
170 int get_buffer (uint32_t address,
const char*& ptr, uint32_t& length)
const;
171 void update_buffer (uint32_t address,
const uint8_t* buff, uint32_t length);
174 uint32_t
get_word (uint32_t address);
175 void update_word (uint32_t address, uint32_t data);
178 uint64_t
get_long (uint32_t address);
179 void update_long (uint32_t address, uint64_t data);
187 void update_string (uint32_t address,
const char* data,
int len = -1);
195 template <
typename T>
201 unsigned char u8[
sizeof(T)];
206 for (
size_t k = 0; k <
sizeof(T); k++)
207 dest.u8[k] = source.u8[
sizeof(T) - k - 1];
212 tsRegDefinition*
reg_def (
const char *name)
const;
213 tsRegDefinition*
reg_def (uint64_t address,
bool sloppy =
false)
const;
214 tsRegDefinition*
next_reg(uint64_t address)
const;
216 uint32_t
reg_addr (
const char *name);
218 std::string
reg_name (uint32_t address);
220 bool reg_rd (uint32_t address);
221 bool reg_wr (uint32_t address);
223 bool reg_a_rd (uint32_t address, int16_t size);
224 bool reg_a_wr (uint32_t address, int16_t size);
228 static std::string
format_memdump (uint8_t* pbuff, uint16_t length);
248 template <
typename ValueType, teRegType RegEnum>
252 if (preg != NULL && preg->
type == RegEnum)
254 memcpy(&retv, &(preg->
numData),
sizeof(ValueType));
265 template <
typename ValueType, teRegType RegEnum>
272 memcpy(&uitemp, &data,
sizeof(ValueType));
273 memcpy(&(preg->
numData), &uitemp,
sizeof(ValueType));
287 template <
typename ValueType, teRegType RegEnum,
typename UpdateMsg>
292 if (preg != NULL && preg->
type == RegEnum)
295 memcpy(&old_value, &(preg->
numData),
sizeof(ValueType));
298 old_value = swap_endian<ValueType>(old_value);
302 UpdateMsg *regMsg =
new UpdateMsg (&pdef, old_value, data);
319 regMsg->m_fixval = swap_endian<ValueType>(regMsg->m_fixval);
321 update_value<ValueType, RegEnum>(address, regMsg->m_fixval);
323 retv = regMsg->m_status;
332 std::map<uint64_t, tsRegDefinition*> maRegisters;
336 struct timespec m_ts_resettime;
441 const uint32_t
VERSION_ADDR = 0x0000
'0000; //!< This register indicates the version of the GigE Vision specification implemented by this device. Version 1.0 of this specification shall return 0x00010000.
442 const uint32_t DEVICE_MODE_ADDR = 0x0000'0004;
443 const uint32_t
DEVICE_MAC_HIGH0_ADDR = 0x0000
'0008; //!< This register stores the MAC address (upper 16-bit) of the given network interface.
444 const uint32_t DEVICE_MAC_LOW0_ADDR = 0x0000'000C;
445 const uint32_t
NET_IFACE_CAPABILITY0_ADDR = 0x0000
'0010; //!< This register indicates the IP configuration scheme supported on the given network interface. Multiple schemes can be supported simultaneously.
446 const uint32_t NET_IFACE_CONFIG0_ADDR = 0x0000'0014;
447 const uint32_t
CURRENT_IP_ADDR0_ADDR = 0x0000
'0024; //!< This register reports the IP address for the given network interface once it has been configured.
448 const uint32_t CURRENT_SUBNET0_ADDR = 0x0000'0034;
449 const uint32_t
CURRENT_GATEWAY0_ADDR = 0x0000
'0044; //!< This register indicates the default gateway IP address to be used on the given network interface.
450 const uint32_t MANUFACTURER_NAME_ADDR = 0x0000'0048;
451 const uint32_t
MODEL_NAME_ADDR = 0x0000
'0068; //!< This registers stores a string containing the device model name. This string uses the character set indicated in the "string character set" register.
452 const uint32_t DEVICE_VERSION_ADDR = 0x0000'0088;
453 const uint32_t
MANUFACTURER_INFO_ADDR = 0x0000
'00A8; //!< This register stores a string containing additional manufacturer-specific information about the device. This string uses the character set indicated in the "string character set" register.
454 const uint32_t SERIAL_NUMBER_ADDR = 0x0000'00D8;
455 const uint32_t
USER_NAME_ADDR = 0x0000
'00E8; //!< String providing the device name.
456 const uint32_t FIRST_URL_ADDR = 0x0000'0200;
457 const uint32_t
SECOND_URL_ADDR = 0x0000
'0400; //!< This register stores the second URsi_meL to the XML device description file. This URL is an alternative if the application was unsuccessful to retrieve the device description file using the first URL. This string uses the character set indicated in the "string character set" register.
458 const uint32_t NB_INTERFACES_ADDR = 0x0000'0600;
459 const uint32_t
PERSISTENT_IP_ADDR0_ADDR = 0x0000
'064C; //!< This register indicates the Persistent IP address for this network interface. It is only used when the device boots with the Persistent IP configuration scheme.
460 const uint32_t PERSISTENT_SUBNET0_ADDR = 0x0000'065C;
461 const uint32_t
PERSISTENT_GATEWAY0_ADDR = 0x0000
'066C; //!< This register indicates the persistent default gateway for this network interface. It is only used when the device boots with the Persistent IP configuration scheme.
462 const uint32_t NB_MSG_CHANNELS_ADDR = 0x0000'0900;
463 const uint32_t
NB_STREAM_CHANNELS_ADDR = 0x0000
'0904; //!< This register reports the number of stream channels supported by this device. A device must support at least one stream channel. A device can support up to 512 stream channels.
464 const uint32_t NB_ACTIVE_LINKS_ADDR = 0x0000'0910;
465 const uint32_t
GVSP_CAPABILITY_ADDR = 0x0000
'092C; //!< Bit 1 indicates SCSP availability, bit 2 indicates legacy 16 bit block ID support available. 2-31 reserved.
466 const uint32_t MSG_CAPABILITY_ADDR = 0x0000'0930;
467 const uint32_t
GVCP_CAPABILITY_ADDR = 0x0000
'0934; //!< This register reports the optional GVCP command supported by this device.
468 const uint32_t HEARTBEAT_TIMEOUT_ADDR = 0x0000'0938;
469 const uint32_t
TIMESTAMP_FREQ_HIGH_ADDR = 0x0000
'093C; //!< This register indicates the number of timestamp tick during 1 second. This corresponds to the timestamp frequency in Hertz. Upper 32-bit.
470 const uint32_t TIMESTAMP_FREQ_LOW_ADDR = 0x0000'0940;
471 const uint32_t
TIMESTAMP_CTRL_ADDR = 0x0000
'0944; //!< This register is used to control the timestamp counter.
472 const uint32_t TIMESTAMP_VALUE_HIGH_ADDR = 0x0000'0948;
473 const uint32_t
TIMESTAMP_VALUE_LOW_ADDR = 0x0000
'094C; //!< This register reports the latched value of the timestamp counter. It is necessary to latch the 64-bit timestamp value to guaranty its integrity when performing the two 32-bit read accesses to retrieve the higher and lower 32-bit portions. Lower 32-bit.
474 const uint32_t GVCP_CONFIGURATION_ADDR = 0x0000'0954;
475 const uint32_t
PENDING_TIMEOUT_ADDR = 0x0000
'0958; //!< Pending Timeout to report the longest GVCP command execution time before issuing a PENDING_ACK. If PENDING_ACK is not supported, then this is the worst-case execution time before command completion.
476 const uint32_t CTRL_SWITCHOVER_KEY_ADDR = 0x0000'095C;
478 const uint32_t PHY_CAPABILITY_ADDR = 0x0000'0964;
479 const uint32_t
PHY_CONFIG_ADDR = 0x0000
'0968; //!< Indicates the currently active physical link configuration
480 const uint32_t IEEE_1588_STATUS_ADDR = 0x0000'096C;
481 const uint32_t
SCHEDULED_ACTION_Q_SIZE_ADDR = 0x0000
'0970; //!< Indicates the number of Scheduled Action Commands that can be queued (size of the queue).
482 const uint32_t CCP_ADDR = 0x0000'0A00;
483 const uint32_t
PA_PORT_ADDR = 0x0000
'0A04; //!< UDP source port of the control channel of the primary application.
484 const uint32_t PA_IP_ADDR = 0x0000'0A14;
485 const uint32_t
MCP_ADDR = 0x0000
'0B00; //!< This register provides port information about the message channel. The message channel is activated when the host_port field is different from 0. Otherwise, the channel is closed.
486 const uint32_t MCDA_ADDR = 0x0000'0B10;
487 const uint32_t
MCTT_ADDR = 0x0000
'0B14; //!< This register provides the transmission timeout value in milliseconds. This indicates the amount of time to wait for acknowledge after a message is sent on the message channel before timeout.
488 const uint32_t MCRC_ADDR = 0x0000'0B18;
489 const uint32_t
MCSP_ADDR = 0x0000
'0B1C; //!< Message Channel Source Port.
490 const uint32_t SCP0_ADDR = 0x0000'0D00;
491 const uint32_t
SCPS0_ADDR = 0x0000
'0D04; //!< This register indicates the packet size in bytes for this stream channel. This is the total packet size, including all headers (Ethernet, IP, UDP and GVSP). It also provides a way to set the IP header "do not fragment" bit and to send stream test packet to the application.
492 const uint32_t SCPD0_ADDR = 0x0000'0D08;
493 const uint32_t
SCDA0_ADDR = 0x0000
'0D18; //!< This register indicates the destination IP address for this stream channel.
494 const uint32_t SCSP0_ADDR = 0x0000'0D1C;
495 const uint32_t
SCC0_ADDR = 0x0000
'0D20; //!< First Stream Channel Capability register.
496 const uint32_t SCCFG0_ADDR = 0x0000'0D24;
499 // GenCP Technology Agnostic Boostrap Registers.
500 const uint32_t ABRM_GENCP_VERSION_ADDR = 0x00000;
501 const uint32_t ABRM_MANUFACTURER_NAME_ADDR = 0x00004;
502 const uint32_t ABRM_MODEL_NAME_ADDR = 0x00044;
503 const uint32_t ABRM_FAMILY_NAME_ADDR = 0x00084;
504 const uint32_t ABRM_DEVICE_VERSION_ADDR = 0x000C4;
505 const uint32_t ABRM_MANUFACTURER_INFO_ADDR = 0x00104;
506 const uint32_t ABRM_SERIAL_NUMBER_ADDR = 0x00144;
507 const uint32_t ABRM_USER_DEFINED_NAME_ADDR = 0x00184;
508 const uint32_t ABRM_DEVICE_CAPABILITY_ADDR = 0x001C4;
509 const uint32_t ABRM_MAX_DEVICE_RESPONSE_TIME_ADDR = 0x001CC;
510 const uint32_t ABRM_MANIFEST_TABLE_ADDRESS_ADDR = 0x001D0;
511 const uint32_t ABRM_SBRM_ADDRESS_ADDR = 0x001D8;
512 const uint32_t ABRM_DEVICE_CONFIGURATION_ADDR = 0x001E0;
513 const uint32_t ABRM_HEARTBEAT_TIMEOUT_ADDR = 0x001E8;
514 const uint32_t ABRM_MESSAGE_CHANNEL_ID_ADDR = 0x001EC;
515 const uint32_t ABRM_TIMESTAMP_ADDR = 0x001F0;
516 const uint32_t ABRM_TIMESTAMP_LATCH_ADDR = 0x001F8;
517 const uint32_t ABRM_TIMESTAMP_INCREMENT_ADDR = 0x001FC;
518 const uint32_t ABRM_ACCESS_PRIVILEGE_ADDR = 0x00204;
519 const uint32_t ABRM_PROTOCOL_ENDIANESS_ADDR = 0x00208;
520 const uint32_t ABRM_IMPLEMENTATION_ENDIANESS_ADDR = 0x0020C;
521 const uint32_t ABRM_RESERVED_ADDR = 0x00210;
523 // ABRM Device Capability register flags
524 #define GENCP_USER_DEFINED_NAME (1 << 0)
525 #define GENCP_ACCESS_PRIVILEGE (1 << 1)
526 #define GENCP_MESSAGE_CHANNEL (1 << 2)
527 #define GENCP_TIMESTAMP (1 << 3)
528 #define GENCP_STRING_ENCODING_ASCII (0 << 4)
529 #define GENCP_STRING_ENCODING_UTF8 (1 << 4)
530 #define GENCP_STRING_ENCODING_UTF16 (2 << 4)
531 #define GENCP_FAMILY_NAME (1 << 8)
532 #define GENCP_SBRM_SUPPORT (1 << 9)
533 #define GENCP_ENDIANESS_REGISTER (1 << 10)
534 #define GENCP_WRITTEN_LENGTH_FIELD (1 << 11)
535 #define GENCP_MULTIEVENT (1 << 12)
537 const uint64_t U3V_DEVICE_CAPABILITY = GENCP_ENDIANESS_REGISTER | GENCP_USER_DEFINED_NAME | GENCP_FAMILY_NAME | GENCP_TIMESTAMP | GENCP_STRING_ENCODING_ASCII | GENCP_SBRM_SUPPORT | GENCP_WRITTEN_LENGTH_FIELD;
539 // ABRM Device Configuration register flags
540 #define GENCP_CONFIG_HEARTBEAT_ENABLE (1 << 0)
541 #define GENCP_CONFIG_MULTIEVENT_ENABLE (1 << 1)
542 const uint64_t U3V_DEVICE_CONFIGURATION = 0;
545 #define SBRM_TABLE_ADDR (0x10000)
548 const uint32_t U3V_VERSION_ADDR = SBRM_TABLE_ADDR;
549 const uint32_t U3V_CP_CABABILITY_ADDR = SBRM_TABLE_ADDR + 0x04;
550 const uint32_t U3V_CP_CONFIGURATION_ADDR = SBRM_TABLE_ADDR + 0x0C;
551 const uint32_t U3V_MAX_COMMAND_TRANS_ADDR = SBRM_TABLE_ADDR + 0x14;
552 const uint32_t U3V_MAX_ACK_TRANS_ADDR = SBRM_TABLE_ADDR + 0x18;
553 const uint32_t U3V_NB_STRTEAM_ADDR = SBRM_TABLE_ADDR + 0x1C;
554 const uint32_t U3V_SIRM_ADDRESS_ADDR = SBRM_TABLE_ADDR + 0x20;
555 const uint32_t U3V_SIRM_LENGTH_ADDR = SBRM_TABLE_ADDR + 0x28;
556 const uint32_t U3V_EIRM_ADDRESS_ADDR = SBRM_TABLE_ADDR + 0x2C;
557 const uint32_t U3V_EIRM_LENGTH_ADDR = SBRM_TABLE_ADDR + 0x34;
558 const uint32_t U3V_IIDC2_ADDRESS_ADDR = SBRM_TABLE_ADDR + 0x38;
559 const uint32_t U3V_CURRENT_SPEED_ADDR = SBRM_TABLE_ADDR + 0x40;
561 // U3V Control Protocol Capability Register
562 #define U3V_SIRM_AVAILABLE (1 << 0)
563 #define U3V_EIRM_AVAILABLE (1 << 1)
564 #define U3V_IIDC2_AVAILABLE (1 << 2)
566 // U3V SBRM Flags/Defaults
567 #define U3V_CMD_EP_BUFF_SIZE (1020) /* Smaller buffer so that the FX3 doesn't get into an error state with something too large
for it */
584 #define U3V_MAX_HEADER (52)
585 #define U3V_MAX_FOOTER (32)
601 #define GENCP_LITTLE_ENDIAN (0xFFFFFFFF)
606 #define XML_REGS_OFFSET (0x30000)
610 const uint32_t DEVICE_RESET_ADDR = XML_REGS_OFFSET + 0x0000'2100;
613 const uint32_t DEVICE_SOFTWARE_DATE_ADDR = XML_REGS_OFFSET + 0x0000'3008;
616 const uint32_t AQUISITION_FRAMERATE_ADDR = XML_REGS_OFFSET + 0x0000'A000;
618 const uint32_t ROI_WIDTH_ADDR = XML_REGS_OFFSET + 0x0000'A008;
620 const uint32_t ROI_OFFSET_X_ADDR = XML_REGS_OFFSET + 0x0000'A010;
622 const uint32_t ACQUISITION_START_ADDR = XML_REGS_OFFSET + 0x0000'A018;
624 const uint32_t PIXEL_FORMAT_ADDR = XML_REGS_OFFSET + 0x0000'A020;
626 const uint32_t BINNING_HORIZONTAL_ADDR = XML_REGS_OFFSET + 0x0000'A028;
628 const uint32_t REVERSE_X_ADDR = XML_REGS_OFFSET + 0x0000'A030;
630 const uint32_t ACQUISITION_NUM_FRAMES_ADDR = XML_REGS_OFFSET + 0x0000'A038;
631 const uint32_t
ACQUISITION_STOP_ADDR =
XML_REGS_OFFSET + 0x0000
'A03C; //!< SFNC 5.5.4 AquisitionStop - Start/stop image acquisition using the specified acquision mode in the Acquisition Mode register
632 const uint32_t DECIMATION_VERTICAL_ADDR = XML_REGS_OFFSET + 0x0000'A040;
635 const uint32_t BOARD_TEMPERATURE_ADDR = XML_REGS_OFFSET + 0x0000'A048;
637 const uint32_t SHUTTER_MODE_ADDR = XML_REGS_OFFSET + 0x0000'A050;
639 const uint32_t SENSOR_CALIBRATE_ADDR = XML_REGS_OFFSET + 0x0000'A058;
642 const uint32_t CLOCK_SPEED_ADDR = XML_REGS_OFFSET + 0x0000'A060;
646 const uint32_t HOT_PIXEL_CORRECT_ADDR = XML_REGS_OFFSET + 0x0000'A068;
649 const uint32_t BAD_PIXEL_CTRL_MAP_ADDR = XML_REGS_OFFSET + 0x0000'A074;
652 const uint32_t VOLTAGE_SENSOR_VALUE_ADDR = XML_REGS_OFFSET + 0x0000'A07C;
655 const uint32_t DEVICE_TEMPERATURE_ADDR = XML_REGS_OFFSET + 0x0000'A084;
659 const uint32_t SENSOR_WIDTH_ADDR = XML_REGS_OFFSET + 0x0000'A090;
662 const uint32_t FREE_RAM_CFG_ADDR = XML_REGS_OFFSET + 0x0000'A100;
666 // LUT Control SFNC 7.x
667 const uint32_t LUT_SELECTOR_ADDR = XML_REGS_OFFSET + 0x0000'A300;
669 const uint32_t LUT_MAXINDEX_ADDR = XML_REGS_OFFSET + 0x0000'A308;
671 const uint32_t LUT_MAXVALUE_ADDR = XML_REGS_OFFSET + 0x0000'A310;
673 // const uint32_t LUT_VALUEALL_ADDR = XML_REGS_OFFSET + TODO; //<! SFNC LUTValueAll - WIP: How does this work?
675 namespace LUTSelector {
681 eeDeviceSpecific = 10,
685 // Analog Control SFNC 6.x
686 const uint32_t GAIN_SELECTOR_ADDR = XML_REGS_OFFSET + 0x0000'A400;
688 const uint32_t GAIN_AUTO_ADDR = XML_REGS_OFFSET + 0x0000'A408;
690 const uint32_t BLACK_LEVEL_SELECTOR_ADDR = XML_REGS_OFFSET + 0x0000'A410;
692 const uint32_t BLACK_LEVEL_AUTO_ADDR = XML_REGS_OFFSET + 0x0000'A418;
694 const uint32_t WHITE_CLIP_SELECTOR_ADDR = XML_REGS_OFFSET + 0x0000'A420;
696 const uint32_t BALANCE_RATIO_SELECTOR_ADDR = XML_REGS_OFFSET + 0x0000'A428;
698 const uint32_t BALANCE_WHITE_AUTO_ADDR = XML_REGS_OFFSET + 0x0000'A430;
700 const uint32_t BLACK_LEVEL_BIAS_ADDR = XML_REGS_OFFSET + 0x0000'A440;
736 const uint32_t COLOR_TRANSF_ENABLE_ADDR = XML_REGS_OFFSET + 0x0000'A504;
738 const uint32_t COLOR_TRANSF_VALUE_ADDR = XML_REGS_OFFSET + 0x0000'A50C;
764 const uint32_t TRIGGER_MODE_ADDR = XML_REGS_OFFSET + 0x0000'B004;
766 const uint32_t TRIGGER_ACTIVATION_ADDR = XML_REGS_OFFSET + 0x0000'B00C;
797 const uint32_t DIGITAL_IO_LINE_MODE_ADDR = XML_REGS_OFFSET + 0x0000'B104;
799 const uint32_t USER_OUTPUT_SELECTOR_ADDR = XML_REGS_OFFSET + 0x0000'B110;
801 const uint32_t DIGITAL_IO_LINE_STATUS_ADDR = XML_REGS_OFFSET + 0x0000'B118;
804 namespace LineSource {
809 eeExposureActive = 5,
819 const uint32_t INDICATOR_CONTROL_ADDR = XML_REGS_OFFSET + 0x0000'B120;
822 const uint32_t IS_CAMERA_COLOR_ADDR = XML_REGS_OFFSET + 0x0000'B200;
825 const uint32_t SENS_VAL_ADDR = XML_REGS_OFFSET + 0x0000'C004;
827 const uint32_t SENS_WRITE_ADDR = XML_REGS_OFFSET + 0x0000'C00C;
831 // Reusable sensor specific registers. Every camera can use these registers for whatever they need. Registers are int32 unless otherwise mentioned.
832 const uint32_t SENS_SPECIFIC_1_ADDR = XML_REGS_OFFSET + 0x0000'D000;
834 const uint32_t SENS_SPECIFIC_3_ADDR = XML_REGS_OFFSET + 0x0000'D008;
836 const uint32_t SENS_SPECIFIC_5_ADDR = XML_REGS_OFFSET + 0x0000'D010;
838 const uint32_t SENS_SPECIFIC_7_ADDR = XML_REGS_OFFSET + 0x0000'D018;
840 const uint32_t SENS_SPECIFIC_9_ADDR = XML_REGS_OFFSET + 0x0000'D020;
842 const uint32_t SENS_SPECIFIC_11_ADDR = XML_REGS_OFFSET + 0x0000'D064;
844 const uint32_t SENS_SPECIFIC_13_ADDR = XML_REGS_OFFSET + 0x0000'D06C;
846 const uint32_t SENS_SPECIFIC_15_ADDR = XML_REGS_OFFSET + 0x0000'D074;
848 const uint32_t SENS_SPECIFIC_17_ADDR = XML_REGS_OFFSET + 0x0000'D07C;
850 const uint32_t SENS_SPECIFIC_19_ADDR = XML_REGS_OFFSET + 0x0000'D084;
852 const uint32_t SENS_SPECIFIC_21_ADDR = XML_REGS_OFFSET + 0x0000'D08C;
854 const uint32_t SENS_SPECIFIC_23_ADDR = XML_REGS_OFFSET + 0x0000'D094;
856 const uint32_t SENS_SPECIFIC_25_ADDR = XML_REGS_OFFSET + 0x0000'D09C;
858 const uint32_t SENS_SPECIFIC_27_ADDR = XML_REGS_OFFSET + 0x0000'D0A4;
860 const uint32_t SENS_SPECIFIC_29_ADDR = XML_REGS_OFFSET + 0x0000'D0AC;
863 const uint32_t SENS_SPECIFIC_FLOAT_1_ADDR = XML_REGS_OFFSET + 0x0000'D100;
865 const uint32_t SENS_SPECIFIC_FLOAT_3_ADDR = XML_REGS_OFFSET + 0x0000'D108;
867 const uint32_t SENS_SPECIFIC_FLOAT_5_ADDR = XML_REGS_OFFSET + 0x0000'D110;
870 const uint32_t SENS_PEEKPOKE_ADDR_END = XML_REGS_OFFSET + 0x0023'FFFF;
877 //===================================================
878 // Debug Buffer Registers
879 // Dynamically registered by GenICamStatusReporter
880 //===================================================
881 const uint32_t DEBUG_BUFFER_BYTESWRITTEN_ADDR = 0x0030'0000;
885 // Set these addresses correctly.
886 const uint32_t MANIFEST_TABLE_COUNT_ADDR = MANIFEST_TABLE_ADDR;
887 const uint32_t MANIFEST_VER_ADDR = MANIFEST_TABLE_ADDR + 0x08;
888 const uint32_t MANIFEST_TYPE_ADDR = MANIFEST_VER_ADDR + 0x04;
889 const uint32_t MANIFEST_REG_ADDR = MANIFEST_TYPE_ADDR + 0x04;
890 const uint32_t MANIFEST_FILE_SIZE_ADDR = MANIFEST_REG_ADDR + 0x08;
891 const uint32_t MANIFEST_SHA1_ADDR = MANIFEST_FILE_SIZE_ADDR + 0x08;
893 //===================================================
895 // TODO What does this mean?
896 //===================================================
897 const uint32_t BEYOND_MANIFEST_ADDR = 0x20000000;
898 const uint32_t TEST_PENDING_ACK_ADDR = BEYOND_MANIFEST_ADDR;
900 const uint32_t HDMI_START_ADDR = BEYOND_MANIFEST_ADDR + 0x04;
901 const uint32_t HDMI_STOP_ADDR = BEYOND_MANIFEST_ADDR + 0x08;
902 const uint32_t HDMI_OFFSET_X_ADDR = BEYOND_MANIFEST_ADDR + 0x0c;
903 const uint32_t HDMI_OFFSET_Y_ADDR = BEYOND_MANIFEST_ADDR + 0x10;
904 const uint32_t HDMI_WIDTH_ADDR = BEYOND_MANIFEST_ADDR + 0x14;
905 const uint32_t HDMI_HEIGHT_ADDR = BEYOND_MANIFEST_ADDR + 0x18;
906 const uint32_t HDMI_OUTPUT_SEL_ADDR = BEYOND_MANIFEST_ADDR + 0x1c;
907 // const uint32_t HDMI_COLOR_SEL_ADDR = BEYOND_MANIFEST_ADDR + 0x20; This is deprecated
908 const uint32_t HDMI_GAMMA_ADDR = BEYOND_MANIFEST_ADDR + 0x24;
909 const uint32_t HDMI_WBRED_ADDR = BEYOND_MANIFEST_ADDR + 0x28;
910 const uint32_t HDMI_WBGREEN_ADDR = BEYOND_MANIFEST_ADDR + 0x2C;
911 const uint32_t HDMI_WBBLUE_ADDR = BEYOND_MANIFEST_ADDR + 0x30;
912 const uint32_t HDMI_TESTPATEN_ADDR = BEYOND_MANIFEST_ADDR + 0x34;
913 const uint32_t HDMI_BPP_ADDR = BEYOND_MANIFEST_ADDR + 0x38;
916 //===================================================
917 // REGISTER FLAG BIT DEFINITIONS
918 //===================================================
924 const uint32_t GIGE_VERSION_1_2 = 0x00010002; //<! GigE version 1.2 (Not used just for reference)
925 const uint32_t GIGE_VERSION_2_0 = 0x00020000; //<! GigE version 2.0 (We use this one)
931 const uint32_t PIXEL_FORMAT_MONO16 = 0x01100007; //<! 16 bit mono
932 const uint32_t PIXEL_FORMAT_MONO8 = 0x01080001; //<! 8 bit mono
933 const uint32_t PIXEL_FORMAT_MONO12 = 0x01100005; //<! 12 bit mono
934 const uint32_t PIXEL_FORMAT_MONO12P = 0x010C0047; //<! 12 bit mono packed
935 const uint32_t PIXEL_FORMAT_MONO12PACKED = 0x010C0007; //<! 12 bit mono packed
937 const uint32_t PIXEL_FORMAT_BAYERGR8 = 0x01080008;
938 const uint32_t PIXEL_FORMAT_BAYERRG8 = 0x01080009;
939 const uint32_t PIXEL_FORMAT_BAYERGB8 = 0x0108000A;
940 const uint32_t PIXEL_FORMAT_BAYERBG8 = 0x0108000B;
942 const uint32_t PIXEL_FORMAT_BAYERGR16 = 0x0110002E;
943 const uint32_t PIXEL_FORMAT_BAYERRG16 = 0x0110002F;
944 const uint32_t PIXEL_FORMAT_BAYERGB16 = 0x01100030;
945 const uint32_t PIXEL_FORMAT_BAYERBG16 = 0x01100031;
947 const uint32_t PIXEL_FORMAT_BAYERBG12P = 0x010C0053;
948 const uint32_t PIXEL_FORMAT_BAYERGB12P = 0x010C0055;
949 const uint32_t PIXEL_FORMAT_BAYERGR12P = 0x010C0057;
950 const uint32_t PIXEL_FORMAT_BAYERRG12P = 0x010C0059;
952 const uint32_t PIXEL_FORMAT_BAYERGR12PACKED = 0x010C002A;
953 const uint32_t PIXEL_FORMAT_BAYERRG12PACKED = 0x010C002B;
954 const uint32_t PIXEL_FORMAT_BAYERGB12PACKED = 0x010C002C;
955 const uint32_t PIXEL_FORMAT_BAYERBG12PACKED = 0x010C002D;
957 /* 32-bit value layout */
958 /* |31 24|23 16|15 08|07 00| */
959 /* | C| Comp. Layout| Effective Size | Pixel ID | */
962 #define PFNC_CUSTOM 0x80000000
963 /* Component layout */
964 #define PFNC_SINGLE_COMPONENT 0x01000000
965 #define PFNC_MULTIPLE_COMPONENT 0x02000000
966 #define PFNC_COMPONENT_MASK 0x7F000000
968 #define PFNC_OCCUPY1BIT 0x00010000
969 #define PFNC_OCCUPY2BIT 0x00020000
970 #define PFNC_OCCUPY4BIT 0x00040000
971 #define PFNC_OCCUPY8BIT 0x00080000
972 #define PFNC_OCCUPY10BIT 0x000A0000
973 #define PFNC_OCCUPY12BIT 0x000C0000
974 #define PFNC_OCCUPY16BIT 0x00100000
975 #define PFNC_OCCUPY24BIT 0x00180000
976 #define PFNC_OCCUPY30BIT 0x001E0000
977 #define PFNC_OCCUPY32BIT 0x00200000
978 #define PFNC_OCCUPY36BIT 0x00240000
979 #define PFNC_OCCUPY40BIT 0x00280000
980 #define PFNC_OCCUPY48BIT 0x00300000
981 #define PFNC_OCCUPY64BIT 0x00400000
982 #define PFNC_PIXEL_SIZE_MASK 0x00FF0000
983 #define PFNC_PIXEL_SIZE_SHIFT 16
985 #define PFNC_PIXEL_ID_MASK 0x0000FFFF
986 /* Pixel format value dissection helpers */
987 #define PFNC_PIXEL_SIZE(X) ((X & PFNC_PIXEL_SIZE_MASK) >> PFNC_PIXEL_SIZE_SHIFT)
988 #define PFNC_IS_PIXEL_SINGLE_COMPONENT(X) ((X & PFNC_COMPONENT_MASK) == PFNC_SINGLE_COMPONENT)
989 #define PFNC_IS_PIXEL_MULTIPLE_COMPONENT(X) ((X & PFNC_COMPONENT_MASK) == PFNC_MULTIPLE_COMPONENT)
990 #define PFNC_IS_PIXEL_CUSTOM(X) ((X & PFNC_CUSTOM) == PFNC_CUSTOM)
991 #define PFNC_PIXEL_ID(X) (X & PFNC_PIXEL_ID_MASK)
998 const uint32_t PRIVILEGE_EXCLUSIVE = 0x00000001; //!< Exclusive control privilege (read-write)
999 const uint32_t PRIVILEGE_CONTROL = 0x00000002; //!< Control privilege (read-write)
1005 const uint32_t GVCP_CAP_UN = 0x80000000; //<! Device has a user defined name register [O-442cd].
1006 const uint32_t GVCP_CAP_SN = 0x40000000; //<! Device has a serial number register [O-441cd].
1007 const uint32_t GVCP_CAP_HD = 0x20000000; //<! Heart beat can be disabled.
1008 const uint32_t GVCP_CAP_LS = 0x10000000; //<! Device has link speed registers.
1009 const uint32_t GVCP_CAP_CAP = 0x08000000; //<! CCP Application Port and IP address registers are supported.
1010 const uint32_t GVCP_CAP_MT = 0x04000000; //<! Manifest Table is supported.
1011 const uint32_t GVCP_CAP_TD = 0x02000000; //<! Test packet is filled with data from the LFSR generator.
1012 const uint32_t GVCP_CAP_DD = 0x01000000; //<! Device has discovery ack delay registers [O-468cd].
1013 const uint32_t GVCP_CAP_WD = 0x00800000; //<! When Discovery ACK Delay register is supported, this bit indicates that the register is writable.
1014 const uint32_t GVCP_CAP_ES = 0x00400000; //<! Support generation of extended status codes introduces in specification 1.1.
1015 const uint32_t GVCP_CAP_PAS = 0x00200000; //<! Primary application switchover capability is supported.
1016 const uint32_t GVCP_CAP_UA = 0x00100000; //<! Unconditional ACTION_CMD is supported.
1017 const uint32_t GVCP_CAP_PTP = 0x00080000; //<! Support for IEEE 1588 PTP.
1018 const uint32_t GVCP_CAP_ES2 = 0x00040000; //<! Support generation of extended status codes introduces in specification 2.0.
1019 const uint32_t GVCP_CAP_SAC = 0x00020000; //<! Scheduled Action Commands are supported.
1020 const uint32_t GVCP_CAP_A = 0x00000040; //<! ACTION_CMD and ACTION_ACK are supported.
1021 const uint32_t GVCP_CAP_PA = 0x00000020; //<! PENDING_ACK is supported.
1022 const uint32_t GVCP_CAP_ED = 0x00000010; //<! EVENTDATA_CMD and EVENTDATA_ACK are supported.
1023 const uint32_t GVCP_CAP_E = 0x00000008; //<! EVENT_CMD and EVENT_ACK are supported.
1024 const uint32_t GVCP_CAP_PR = 0x00000004; //<! PACKETRESEND_CMD is supported.
1025 const uint32_t GVCP_CAP_W = 0x00000002; //<! WRITEMEM_CMD and WRITEMEM_ACK are supported.
1026 const uint32_t GVCP_CAP_C = 0x00000001; //<! Multiple operations in a single message are supported.
1027 const uint32_t GVCP_CAP_VALUE = GVCP_CAP_UN | GVCP_CAP_SN | GVCP_CAP_CAP | GVCP_CAP_W | GVCP_CAP_PR;
1032 const uint32_t GVsP_CAP_SP = 0x80000000; //<! Indicates the SCSPx registers (stream channel source port) are available for all supported stream channels.
1033 const uint32_t GVsP_CAP_LB = 0x40000000; //<! Indicates this GVSP transmitter or receiver can support 16-bit block_id.
1034 const uint32_t GVsP_CAP_VALUE = GVsP_CAP_SP | GVsP_CAP_LB;
1039 const uint32_t GVsP_CFG_BL = 0x40000000; //<! Enable the 64-bit block_id64 for GVSP.
1044 const uint32_t PHY_CAP_DLAG = 0x00000008; //!< This device supports dynamic link aggregation configuration.
1045 const uint32_t PHY_CAP_SLAG = 0x00000004; //!< This device supports static link aggregation configuration.
1046 const uint32_t PHY_CAP_ML = 0x00000002; //!< This device supports multiple link (ML) configuration.
1047 const uint32_t PHY_CAP_SL = 0x00000001; //!< This device supports single link (SL) configuration.
1053 const uint32_t PHY_CONFIG_DLAG = 3; //!< Dynamic link aggregation configuration.
1054 const uint32_t PHY_CONFIG_SLAG = 2; //!< Static link aggregation configuration.
1055 const uint32_t PHY_CONFIG_ML = 1; //!< Multiple link (ML) configuration.
1056 const uint32_t PHY_CONFIG_SL = 0; //!< Single link (SL) configuration.
1062 const uint32_t DEVMODE_BIGENDIAN = 0x80000000; //<! Big Endian (required).
1063 const uint32_t DEVMODE_ASCII = 0x00000002; //<! ASCII Character set.
1064 const uint32_t DEVMODE_UTF8 = 0x00000001; //<! UTF-8 Character set.
1070 const uint32_t SHUTTERMODE_ROLLING = 0x00000000; //<! Rolling shutter mode.
1071 const uint32_t SHUTTERMODE_GLOBAL = 0x00000001; //<! Global shutter mode.
1077 const uint32_t NET_IFACE_C_PR = 0x80000000; //<! Pause Reception (we don't use
this).
1116 #endif // REGISTERFILE_H
const uint32_t BALANCE_RATIO_ADDR
Definition: RegisterFile.h:697
const uint64_t U3V_CP_CAPABILITY
Definition: RegisterFile.h:576
static const uint32_t cnMAX_IMAGE_WIDTH
These may be dependant on the sensor type, but for now just want a handy place to put them.
Definition: RegisterFile.h:129
const uint32_t SCPS_D
Definition: RegisterFile.h:1089
uint64_t address
the logical address of the register
Definition: RegisterFile.h:153
@ eeBlue
Definition: RegisterFile.h:707
const uint32_t SC_CAP_R
Definition: RegisterFile.h:1099
const uint32_t U3V_REQ_TRAILER_SIZE_ADDR
Definition: RegisterFile.h:592
const uint32_t BINNING_VERTICAL_ADDR
SFNC 4.28 BinningVertical - Number of pixels to bin in the vertical direction.
Definition: RegisterFile.h:627
const uint32_t SENS_SPECIFIC_10_ADDR
Definition: RegisterFile.h:841
const uint32_t USER_OUTPUT_VALUE_ADDR
Definition: RegisterFile.h:800
const uint32_t DEVICE_MAC_HIGH0_ADDR
This register stores the MAC address (upper 16-bit) of the given network interface.
Definition: RegisterFile.h:443
const uint32_t SCPS_P
Definition: RegisterFile.h:1090
const uint32_t SCC0_ADDR
First Stream Channel Capability register.
Definition: RegisterFile.h:495
@ eeOff
Definition: RegisterFile.h:727
std::string reg_name(uint32_t address)
Definition: RegisterFile.cpp:164
const uint32_t U3V_PAYLOAD_SIZE_ADDR
Definition: RegisterFile.h:594
const uint32_t SC_CAP_MZ
Definition: RegisterFile.h:1100
teRegType type
the register type
Definition: RegisterFile.h:156
void show_all_registers()
Definition: RegisterFile.cpp:1283
@ eeFrameStart
Definition: RegisterFile.h:773
const uint32_t GAIN_AUTOBALANCE_ADDR
Definition: RegisterFile.h:689
const uint32_t BOOTSTRAP_REGISTER_SIZE_B
Size of bootstrap register space.
Definition: RegisterFile.h:70
tcObservable * mpObservable
Definition: Observer.h:8
static const uint32_t cnMAX_IMAGE_HEIGHT
Definition: RegisterFile.h:130
const uint32_t U3V_NB_STREAMS
Definition: RegisterFile.h:580
@ eeFrameActive
Definition: RegisterFile.h:775
const uint32_t VOLTAGE_SENSOR_SELECT_ADDR
Selects which ADC / voltage sensor to read back.
Definition: RegisterFile.h:651
const uint32_t MANUFACTURER_REGISTER_SIZE_B
Size of manufacturer register space.
Definition: RegisterFile.h:69
const GEV_STATUS GEV_STATUS_INVALID_ADDRESS
An attempt was made to access a non existent address space location.
Definition: GigE.h:28
@ eeFrameEnd
Definition: RegisterFile.h:774
const uint32_t SENS_REG_ADDR_ADDR
Definition: RegisterFile.h:824
const uint32_t SC_CAP_VALUE
Definition: RegisterFile.h:1105
tcRegUpdateTypeMsg< std::string > tcRegUpdateStringMsg
Definition: RegisterFile.h:420
@ eeAnalogRed
Definition: RegisterFile.h:712
const uint32_t SENS_SPECIFIC_4_ADDR
Definition: RegisterFile.h:835
std::string get_string(uint32_t address)
Definition: RegisterFile.cpp:937
uint32_t reg_addr(const char *name)
Definition: RegisterFile.cpp:136
void update_float(uint32_t address, float data)
Lean and mean write to shadow. Does not ripple through observers.
Definition: RegisterFile.cpp:906
const uint32_t ACQUISITION_STOP_ADDR
SFNC 5.5.4 AquisitionStop - Start/stop image acquisition using the specified acquision mode in the Ac...
Definition: RegisterFile.h:631
const uint32_t EXPOSURE_TIME_ADDR
SFNC 5.7.4 ExposureTime - This register sets the Exposure time (uS).
Definition: RegisterFile.h:617
@ eeGain02
Definition: RegisterFile.h:749
const uint32_t BLACK_LEVEL_ADDR
Definition: RegisterFile.h:691
const uint32_t GVSP_CONFIGURATION_ADDR
This register enables the 64-bit block_id64 for GVSP.
Definition: RegisterFile.h:477
const uint32_t NB_STREAM_CHANNELS_ADDR
This register reports the number of stream channels supported by this device. A device must support a...
Definition: RegisterFile.h:463
const uint32_t TIMESTAMP_VALUE_LOW_ADDR
This register reports the latched value of the timestamp counter. It is necessary to latch the 64-bit...
Definition: RegisterFile.h:473
const uint32_t LUT_INDEX_ADDR
Definition: RegisterFile.h:670
uint64_t dfltlval
storage for 8 byte integer (signed or unsigned)
Definition: RegisterFile.h:137
void * dfltrptr
storage for RAW binary buffer pointer
Definition: RegisterFile.h:138
tsRegDefinition * next_reg(uint64_t address) const
Definition: RegisterFile.cpp:106
const uint32_t U3V_SIRM_TABLE_ADDR
Definition: RegisterFile.h:587
GEV_STATUS set_value(uint32_t address, ValueType data)
Definition: RegisterFile.h:288
void update_payload_size()
This function is called whenever a register has been changed that will effect the payload size.
Definition: RegisterFile.cpp:1035
T m_newval
This is the new value that we are trying to change to.
Definition: RegisterFile.h:380
Definition: RegisterFile.h:702
@ eeOffset0
Definition: RegisterFile.h:756
teRegType m_regtype
Definition: RegisterFile.h:364
tcRegUpdateTypeMsg< void * > tcRegUpdateBufferMsg
Definition: RegisterFile.h:432
const uint32_t U3V_MAX_LEADER_ADDR
Definition: RegisterFile.h:593
const uint32_t SQRT_COMPRESS_ADDR
Definition: RegisterFile.h:644
float get_float(uint32_t address)
Definition: RegisterFile.cpp:891
@ eeGain10
Definition: RegisterFile.h:750
const uint32_t EDGE_DETECTION_ADDR
Definition: RegisterFile.h:636
const uint32_t TIMESTAMP_CTRL_L
Flags for TIMESTAMP_CTRL Register.
Definition: RegisterFile.h:1111
const uint32_t PA_PORT_ADDR
UDP source port of the control channel of the primary application.
Definition: RegisterFile.h:483
@ eeGain00
Definition: RegisterFile.h:747
const uint32_t SENS_SPECIFIC_8_ADDR
Definition: RegisterFile.h:839
@ eeFrameBurstStart
Definition: RegisterFile.h:776
bool reg_a_rd(uint32_t address, int16_t size)
Is the specified register address space readable.
Definition: RegisterFile.cpp:275
void update_value(uint64_t address, ValueType data)
Definition: RegisterFile.h:266
const uint32_t COLOR_TRANSF_VALUE_SELECTOR_ADDR
Definition: RegisterFile.h:737
const uint32_t U3V_REQ_LEADER_SIZE_ADDR
Definition: RegisterFile.h:591
eeTriggerSelected
Definition: RegisterFile.h:769
int addRegister(tsRegDefinition *pdef)
Definition: RegisterFile.cpp:443
@ eeRegFloat
Single precision interface.
Definition: RegisterFile.h:113
const uint32_t GVCP_CAPABILITY_ADDR
This register reports the optional GVCP command supported by this device.
Definition: RegisterFile.h:467
@ eeGain20
Definition: RegisterFile.h:753
@ eeFrameBurstEnd
Definition: RegisterFile.h:777
const uint32_t SENSOR_GAINMODE_ADDR
Definition: RegisterFile.h:638
@ eeGreen
Definition: RegisterFile.h:706
const uint32_t GVSP_CAPABILITY_ADDR
Bit 1 indicates SCSP availability, bit 2 indicates legacy 16 bit block ID support available....
Definition: RegisterFile.h:465
tuDataType numData
union for literal value of register (int, float, raw pointer)
Definition: RegisterFile.h:159
const uint32_t SENS_SPECIFIC_26_ADDR
Definition: RegisterFile.h:857
@ eeGain12
Definition: RegisterFile.h:752
const uint32_t BAD_PIXEL_CTRL_ADDR
Definition: RegisterFile.h:648
void latch_timestamp()
Definition: RegisterFile.cpp:1111
bool reg_a_wr(uint32_t address, int16_t size)
Is the specified register address space writeable.
Definition: RegisterFile.cpp:306
void sync_manifest_table(const std::string &arXmlFile, const uint64_t fpga_version)
Definition: RegisterFile.cpp:1162
bool m_handled
Definition: RegisterFile.h:366
const uint32_t FAN_CONTROL_ADDR
control for FAN
Definition: RegisterFile.h:820
const uint32_t U3V_PAYLOAD_FINAL1_ADDR
Definition: RegisterFile.h:596
@ eeY
Definition: RegisterFile.h:708
Definition: RegisterFile.h:785
uint32_t dfltnval
storage for 4 byte integer (signed or unsigned)
Definition: RegisterFile.h:135
void initialize(tcSensorBoard *apSensor, tcPayloadSetter *apIface)
Definition: RegisterFile.cpp:610
const uint32_t NET_IFACE_C_L
Definition: RegisterFile.h:1079
const tcRegisterFile::tsRegDefinition * m_reg_def
Definition: RegisterFile.h:363
const uint32_t DEVICE_FPGA_VERSION_ADDR
Definition: RegisterFile.h:612
uint32_t m_address
Definition: RegisterFile.h:365
const uint32_t SENS_PEEKPOKE_ADDR
Definition: RegisterFile.h:869
const uint32_t SENSOR_HEIGHT_ADDR
SFNC 4.3 SensorHeight - Effective height of sensor in pixels.
Definition: RegisterFile.h:660
#define XML_REGS_OFFSET
Definition: RegisterFile.h:606
@ eeAcquisitionActive
Definition: RegisterFile.h:772
bool reg_a_valid(uint32_t address, int16_t size)
Is the specified register address space valid.
Definition: RegisterFile.cpp:244
tsRegDefinition * reg_def(const char *name) const
Definition: RegisterFile.cpp:48
void sync_user_name(uint64_t anAddress)
Definition: RegisterFile.cpp:388
const uint32_t SENS_SPECIFIC_24_ADDR
Definition: RegisterFile.h:855
@ eeDigitalBlue
Definition: RegisterFile.h:721
@ eeAnalogGreen
Definition: RegisterFile.h:713
@ eeDigitalV
Definition: RegisterFile.h:724
@ eeExposureEnd
Definition: RegisterFile.h:781
int get_buffer(uint32_t address, const char *&ptr, uint32_t &length) const
Definition: RegisterFile.cpp:748
Definition: RegisterFile.h:740
uint32_t get_word(uint32_t address)
Definition: RegisterFile.cpp:846
Definition: RegisterFileObserver.h:18
const uint32_t ACQUISITION_MODE_ADDR
SFNC 5.5.2 AcquisitionMode - Controls acquisition mode.
Definition: RegisterFile.h:623
const GEV_STATUS GEV_STATUS_SUCCESS
Requested operation was completed successfully.
Definition: GigE.h:25
bool wraccess
whether the register may be written by an external interface
Definition: RegisterFile.h:158
const uint32_t U3V_REQ_PAYLOAD_SIZE_ADDR
Definition: RegisterFile.h:590
const uint32_t SCHEDULED_ACTION_Q_SIZE_ADDR
Indicates the number of Scheduled Action Commands that can be queued (size of the queue).
Definition: RegisterFile.h:481
const uint32_t XML_FILE_SIZE_B
Size allocated for XML file in register space.
Definition: RegisterFile.h:71
const struct timespec * get_resettime()
Definition: RegisterFile.h:237
const uint32_t U3V_SIRM_CTRL_ADDR
Definition: RegisterFile.h:589
const uint32_t SCPS_PKT_SIZE_MASK
Definition: RegisterFile.h:1091
GEV_STATUS m_status
Definition: RegisterFile.h:367
@ eeV
Definition: RegisterFile.h:710
void * mpMessage
Definition: Observer.h:9
const uint32_t TIMESTAMP_FREQ_HIGH_ADDR
This register indicates the number of timestamp tick during 1 second. This corresponds to the timesta...
Definition: RegisterFile.h:469
Definition: Observable.h:7
eeAnalogAuto
Definition: RegisterFile.h:726
const uint32_t SENSOR_TEMPERATURE_ADDR
Definition: RegisterFile.h:634
void reset_timestamp()
Definition: RegisterFile.cpp:1214
const uint32_t SENS_SPECIFIC_FLOAT_4_ADDR
Definition: RegisterFile.h:866
const uint32_t VERSION_ADDR
This register indicates the version of the GigE Vision specification implemented by this device....
Definition: RegisterFile.h:441
const uint32_t SC_CAP_PRD
Definition: RegisterFile.h:1101
@ eeRisingEdge
Definition: RegisterFile.h:787
@ eeLevelLow
Definition: RegisterFile.h:791
const uint32_t NET_IFACE_C_P
Definition: RegisterFile.h:1081
const uint32_t SENS_SPECIFIC_FLOAT_2_ADDR
Definition: RegisterFile.h:864
void notifyChange(tsUpdate asMessage)
Definition: Observable.cpp:17
ValueType get_value(uint64_t address)
Definition: RegisterFile.h:249
const uint32_t SCPS_MASK
Definition: RegisterFile.h:1092
uint32_t m_length
Definition: RegisterFile.h:368
@ eeContinuous
Definition: RegisterFile.h:729
const uint64_t U3V_CP_CONFIGURATION
Definition: RegisterFile.h:577
const uint32_t TOTAL_REGISTER_SIZE_B
Definition: RegisterFile.h:72
const uint32_t GAIN_ADDR
Definition: RegisterFile.h:687
const uint32_t SENS_SPECIFIC_12_ADDR
Definition: RegisterFile.h:843
const uint32_t ROI_HEIGHT_ADDR
SFNC 4.19 Height - This register sets the height of the ROI.
Definition: RegisterFile.h:619
const uint32_t PHY_CONFIG_ADDR
Indicates the currently active physical link configuration.
Definition: RegisterFile.h:479
Definition: RegisterFile.h:151
tcRegUpdateTypeMsg(const tcRegisterFile::tsRegDefinition *preg, T oldval, T newval)
Definition: RegisterFile.h:375
const uint32_t U3V_VERSION
Definition: RegisterFile.h:575
const uint32_t SENS_SPECIFIC_16_ADDR
Definition: RegisterFile.h:847
const uint32_t SC_CAP_US
Definition: RegisterFile.h:1103
const uint32_t REVERSE_Y_ADDR
SFNC 4.34 ReverseY - "Bool" that flips Y when true.
Definition: RegisterFile.h:629
GEV_STATUS set_buffer(uint32_t address, const uint8_t *buff, uint32_t length)
Definition: RegisterFile.cpp:798
const uint32_t MANIFEST_TABLE_ADDR
Definition: RegisterFile.h:497
const uint32_t U3V_PAYLOAD_COUNT_ADDR
Definition: RegisterFile.h:595
const uint32_t CURRENT_GATEWAY0_ADDR
This register indicates the default gateway IP address to be used on the given network interface.
Definition: RegisterFile.h:449
@ eeBigEndian
Definition: RegisterFile.h:143
T m_oldval
This is the old value before we decided to try and change it.
Definition: RegisterFile.h:379
bool reg_wr(uint32_t address)
Is the specified register address writeable.
Definition: RegisterFile.cpp:221
static const uint32_t cnNOADDRESS
Definition: RegisterFile.h:125
const uint32_t SENS_SPECIFIC_22_ADDR
Definition: RegisterFile.h:853
@ eeLittleEndian
Definition: RegisterFile.h:142
Definition: RegisterFile.h:134
const uint32_t TEST_PATTERN_ADDR
Definition: RegisterFile.h:641
const uint32_t NET_IFACE_C_MASK
Definition: RegisterFile.h:1082
Definition: RegisterFile.h:372
void update_word(uint32_t address, uint32_t data)
Lean and mean write to shadow. Does not ripple through observers.
Definition: RegisterFile.cpp:861
const uint32_t ROI_OFFSET_Y_ADDR
SFNC 4.21 OffsetY - This register stores the starting row of the ROI.
Definition: RegisterFile.h:621
const uint32_t DEVICE_FIRMWARE_VERSION_ADDR
Definition: RegisterFile.h:609
@ eeRegUint32
Generic 32-bit unsigned integer interface (for endian management)
Definition: RegisterFile.h:112
const uint32_t PENDING_TIMEOUT_ADDR
Pending Timeout to report the longest GVCP command execution time before issuing a PENDING_ACK....
Definition: RegisterFile.h:475
const uint32_t TIMESTAMP_CTRL_ADDR
This register is used to control the timestamp counter.
Definition: RegisterFile.h:471
@ eeU
Definition: RegisterFile.h:709
const uint32_t SC_CAP_AIT
Definition: RegisterFile.h:1102
const uint32_t U3V_MAX_ACK_LEN
Definition: RegisterFile.h:579
@ eeAnalogU
Definition: RegisterFile.h:716
const uint32_t SECOND_URL_ADDR
This register stores the second URsi_meL to the XML device description file. This URL is an alternati...
Definition: RegisterFile.h:457
const uint32_t SENS_SPECIFIC_2_ADDR
Definition: RegisterFile.h:833
@ eeRGBtoRGB
Definition: RegisterFile.h:742
eeColorTransfSelected
Definition: RegisterFile.h:741
@ eeLevelHigh
Definition: RegisterFile.h:790
@ eeAcquisitionStart
Definition: RegisterFile.h:770
const uint32_t LUT_VALUE_ADDR
Definition: RegisterFile.h:672
eeAnalogSelected
Definition: RegisterFile.h:703
const uint32_t U3V_PAYLOAD_FINAL2_ADDR
Definition: RegisterFile.h:597
const uint32_t MODEL_NAME_ADDR
This registers stores a string containing the device model name. This string uses the character set i...
Definition: RegisterFile.h:451
GEV_STATUS set_word(uint32_t address, uint32_t data)
Definition: RegisterFile.cpp:877
const uint32_t DEVICE_TEMPERATURE_SELECT_ADDR
SFNC 3.60 DeviceTemperatureSelector.
Definition: RegisterFile.h:654
int reg_size(uint32_t address)
Definition: RegisterFile.cpp:150
const uint32_t MCTT_ADDR
This register provides the transmission timeout value in milliseconds. This indicates the amount of t...
Definition: RegisterFile.h:487
@ eeRegString
A string interface (for variable size / null termination)
Definition: RegisterFile.h:115
Definition: PayloadSetter.h:11
const uint32_t DIGITAL_IO_LINE_SOURCE_ADDR
Definition: RegisterFile.h:798
@ eeOffset1
Definition: RegisterFile.h:757
GEV_STATUS set_long(uint32_t address, uint64_t data)
Definition: RegisterFile.cpp:377
const uint32_t SCPS_F
Flags for SCPS0 Register.
Definition: RegisterFile.h:1088
Definition: RegisterFile.h:768
@ eeExposureActive
Definition: RegisterFile.h:782
float dfltfval
storage for 4 byte single precision float
Definition: RegisterFile.h:136
@ eeRGBtoYUV
Definition: RegisterFile.h:743
@ eeDigitalU
Definition: RegisterFile.h:723
GEV_STATUS set_float(uint32_t address, float data)
Definition: RegisterFile.cpp:922
@ eeDigitalAll
Definition: RegisterFile.h:718
const uint32_t PERSISTENT_IP_ADDR0_ADDR
This register indicates the Persistent IP address for this network interface. It is only used when th...
Definition: RegisterFile.h:459
const uint32_t MANUFACTURER_INFO_ADDR
This register stores a string containing additional manufacturer-specific information about the devic...
Definition: RegisterFile.h:453
const uint32_t DIGITAL_IO_LINE_SELECT_ADDR
Definition: RegisterFile.h:796
const uint32_t SC_CAP_EC
Definition: RegisterFile.h:1104
const uint32_t EXPOSURE_TIME_SELECT_ADDR
SFNC 5.7.3 ExposureTimeSelector - This optional register allows selecting different exposure times (s...
Definition: RegisterFile.h:657
const uint32_t SENS_READ_ADDR
Definition: RegisterFile.h:826
Definition: CameraTypes.h:7
void update_buffer(uint32_t address, const uint8_t *buff, uint32_t length)
Definition: RegisterFile.cpp:772
const uint32_t SENS_SPECIFIC_14_ADDR
Definition: RegisterFile.h:845
static const uint32_t cnMAX_PAYLOAD_SIZE
Definition: RegisterFile.h:131
const uint32_t TRIGGER_SELECTOR_ADDR
Definition: RegisterFile.h:763
const uint32_t SCPS0_ADDR
This register indicates the packet size in bytes for this stream channel. This is the total packet si...
Definition: RegisterFile.h:491
bool reg_rd(uint32_t address)
Is the specified register address readable.
Definition: RegisterFile.cpp:208
teEndianness
Definition: RegisterFile.h:141
@ eeRed
Definition: RegisterFile.h:705
const uint32_t CURRENT_IP_ADDR0_ADDR
This register reports the IP address for the given network interface once it has been configured.
Definition: RegisterFile.h:447
const uint32_t TRIGGER_SOURCE_ADDR
Definition: RegisterFile.h:765
const uint32_t INTERNAL_EXP_MODE_ADDR
Definition: RegisterFile.h:664
Definition: RegisterFile.h:121
const uint32_t SENS_SPECIFIC_30_ADDR
Definition: RegisterFile.h:861
static T swap_endian(T u)
Definition: RegisterFile.h:196
const uint32_t SENS_SPECIFIC_20_ADDR
Definition: RegisterFile.h:851
@ eeAnalogBlue
Definition: RegisterFile.h:714
#define U3V_CMD_EP_BUFF_SIZE
Definition: RegisterFile.h:567
@ eeAnyEdge
Definition: RegisterFile.h:789
@ eeLineStart
Definition: RegisterFile.h:779
@ eeGain21
Definition: RegisterFile.h:754
const uint32_t MCP_ADDR
This register provides port information about the message channel. The message channel is activated w...
Definition: RegisterFile.h:485
const uint32_t PERSISTENT_GATEWAY0_ADDR
This register indicates the persistent default gateway for this network interface....
Definition: RegisterFile.h:461
uint64_t get_long(uint32_t address)
Definition: RegisterFile.cpp:366
const uint32_t DIGITAL_IO_LINE_INVERTER_ADDR
Definition: RegisterFile.h:802
const char * name
the logical name of the register
Definition: RegisterFile.h:154
uint32_t size
the size of the register in bytes
Definition: RegisterFile.h:155
tcRegUpdateTypeMsg< uint32_t > tcRegUpdateUint32Msg
Definition: RegisterFile.h:396
@ eeFrameBurstActive
Definition: RegisterFile.h:778
void check_and_update_payload_size(uint64_t address)
Definition: RegisterFile.cpp:1016
@ eeRegNoType
Generic Register interface (an array of Binary Data)
Definition: RegisterFile.h:111
@ eeAnalogY
Definition: RegisterFile.h:715
@ eeDeviceSpecific
Definition: RegisterFile.h:744
const uint32_t LUT_ENABLE_ADDR
Definition: RegisterFile.h:668
const uint32_t SENS_SPECIFIC_28_ADDR
Definition: RegisterFile.h:859
const uint32_t NET_IFACE_C_D
Definition: RegisterFile.h:1080
static bool instanceMade()
Definition: RegisterFile.cpp:383
const uint32_t U3V_MAX_TRAILER_SIZE_ADDR
Definition: RegisterFile.h:598
bool rdaccess
whether the register may be read by an external interface
Definition: RegisterFile.h:157
static tcRegisterFile * getInstance(tsRegDefinition *apRegs=NULL, bool abBigEndian=true)
Definition: RegisterFile.cpp:355
const uint32_t DEVICE_FX3_DATE_ADDR
Definition: RegisterFile.h:614
GEV_STATUS set_string(uint32_t address, const char *data)
Definition: RegisterFile.cpp:980
@ eeAnalogAll
Definition: RegisterFile.h:711
bool reg_valid(uint32_t address)
Is the specified register address valid.
Definition: RegisterFile.cpp:195
const uint32_t MCSP_ADDR
Message Channel Source Port.
Definition: RegisterFile.h:489
void sharedInit()
Definition: RegisterFile.cpp:411
uint16_t GEV_STATUS
Definition: GigE.h:24
@ eeRegUint64
Generic 64-bit unsigned integer interface (for endian management)
Definition: RegisterFile.h:114
const uint32_t SCDA0_ADDR
This register indicates the destination IP address for this stream channel.
Definition: RegisterFile.h:493
static std::string format_memdump(uint8_t *pbuff, uint16_t length)
This function is for debugging. It formats a multiline string.
Definition: RegisterFile.cpp:1295
const uint32_t SENS_SPECIFIC_18_ADDR
Definition: RegisterFile.h:849
@ eeOffset2
Definition: RegisterFile.h:758
const uint32_t U3V_MAX_CMD_LEN
Definition: RegisterFile.h:578
tcRegUpdateTypeMsg< float > tcRegUpdateFloatMsg
Definition: RegisterFile.h:408
@ eeDeviceSpecific
Definition: RegisterFile.h:730
const uint32_t PAYLOAD_SIZE_ADDR
SFNC 25.2.5 PayloadSize - Size of frame without headers.
Definition: RegisterFile.h:625
tcRegUpdateTypeMsg< uint64_t > tcRegUpdateUint64Msg
Definition: RegisterFile.h:384
Definition: SensorBoard.h:40
void update_long(uint32_t address, uint64_t data)
Lean and mean write to shadow. Does not ripple through observers.
Definition: RegisterFile.cpp:372
tcRegisterFile(tsRegDefinition *apRegs, bool abBigEndian)
Definition: RegisterFile.cpp:526
const uint32_t USER_NAME_ADDR
String providing the device name.
Definition: RegisterFile.h:455
const uint32_t DEBUG_BUFFER_ADDR
Definition: RegisterFile.h:882
teRegType
Specifies what kind of data a register holds.
Definition: RegisterFile.h:110
const uint32_t XML_FILE_ADDR
This is where the XML file is loaded into memory.
Definition: RegisterFile.h:875
@ eeAcquisitionEnd
Definition: RegisterFile.h:771
const uint32_t SC_CAP_BE
Flags for SCC0 Register.
Definition: RegisterFile.h:1098
#define U3V_SIRM_AVAILABLE
Definition: RegisterFile.h:562
int get_array(uint32_t address, uint8_t *buff, uint16_t length) const
Retrieve RAW memory from register space.
Definition: RegisterFile.cpp:668
const uint32_t U3V_SIRM_LENGTH
Definition: RegisterFile.h:583
const uint32_t WHITE_CLIP_ADDR
Definition: RegisterFile.h:695
const uint32_t TIMESTAMP_CTRL_R
Definition: RegisterFile.h:1112
@ eeAll
Definition: RegisterFile.h:704
@ eeDigitalRed
Definition: RegisterFile.h:719
const uint32_t U3V_SIRM_INFO_ADDR
Definition: RegisterFile.h:588
@ eeDigitalY
Definition: RegisterFile.h:722
const uint32_t GAMMA_ADDR
Definition: RegisterFile.h:699
eeColorTransfValueSelected
Definition: RegisterFile.h:746
T m_fixval
This is the new value after all the handlers have had a chance verify it. It' may be different than t...
Definition: RegisterFile.h:381
tcRegUpdateMsg(const tcRegisterFile::tsRegDefinition *preg)
Definition: RegisterFile.h:354
std::string dfltsval
used to store the string value of a string register
Definition: RegisterFile.h:160
const uint32_t BLACK_LEVEL_AUTOBALANCE_ADDR
Definition: RegisterFile.h:693
Definition: RegisterFile.h:351
@ eeGain11
Definition: RegisterFile.h:751
const uint32_t COLOR_TRANSF_SELECTOR_ADDR
Definition: RegisterFile.h:735
@ eeOnce
Definition: RegisterFile.h:728
const uint32_t SENS_MIN_FRAME_PERIOD_ADDR
Definition: RegisterFile.h:829
teTriggerActivation
Definition: RegisterFile.h:786
void show_register(const tsRegDefinition *pregdef)
Definition: RegisterFile.cpp:1224
const uint32_t SENS_SPECIFIC_6_ADDR
Definition: RegisterFile.h:837
const uint32_t NET_IFACE_CAPABILITY0_ADDR
This register indicates the IP configuration scheme supported on the given network interface....
Definition: RegisterFile.h:445
@ eeFallingEdge
Definition: RegisterFile.h:788
@ eeExposureStart
Definition: RegisterFile.h:780
@ eeAnalogV
Definition: RegisterFile.h:717
void update_string(uint32_t address, const char *data, int len=-1)
Lean and mean write to shadow. Does not ripple through observers.
Definition: RegisterFile.cpp:956
const uint32_t NET_IFACE_C_PG
Definition: RegisterFile.h:1078
@ eeGain01
Definition: RegisterFile.h:748
@ eeDigitalGreen
Definition: RegisterFile.h:720
@ eeGain22
Definition: RegisterFile.h:755